Lightning Component Wrapper Classes
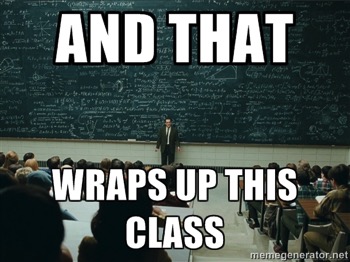
Introduction
Wrapper classes are a common feature of applications built on the Salesforce platform. The class wraps an sObject and some additional data, hence the name wrapper. Typically wrapper classes are used to temporarily associate prpoerties with an sObject that are specific to the current scenario, and that do not make sense to add as fields to the sObject and persist to the database.
In this post I’ll show how you can use wrapper classes in a Lightning component, to wrap an account and a boolean property. This wrapper class allows a user to select one or more account names to view more details of the accounts.
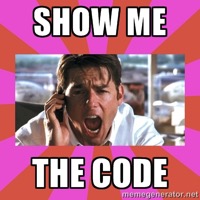
The Apex Controller
My Apex controller makes a couple of methods available to the Lightning component. The first is invoked when the component initialises, and returns a collection of up to 10 accounts, with only the name and Id fields populated:
@AuraEnabled
public static List<Account> GetAccountNames()
{
return [select id, Name from Account limit 10];
}
the second method receives a JSON string representing a list of account ids (the accounts that the user has selected) and retrieves more details for those accounts:
@AuraEnabled
public static List<Account> GetAccountDetails(String idListJSONStr)
{
Type idArrType=Type.forName('List<Id>');
List<Id> ids=(List<Id>) JSON.deserialize(idListJSONStr, idArrType);
return [select id, Name, Industry, Website from Account where id in :ids];
}
Ideally I’d pass the Ids through as an array to this method, but attempting to pass arrays as parameter to Apex controller methods from a Lightning component results in an internal server error. At the time of writing (December 2015) this has been acknowledged as a bug for over a year, so its clearly one that is taking a bit of fixing! For a collection of primitives, the JSON string version works well, but when I need to send an array of complex objects (or sObjects) I usually place these inside a containing Apex class.
The Wrapper Class
I want my wrapper class to be available for use in both my component and in Apex, so that I can use the same one for Lightning and Visualforce. As I’ve written about before, custom apex classes are automatically converted to JavaScript objects for use client side, so to achieve this all I have to do is make the class properties available to the Lightning component via the AuraEnabled annotation:
public with sharing class AccountWrapper
{
@AuraEnabled
public Account acc {get; set;}
@AuraEnabled
public Boolean selected {get; set;}
}
(If I didn’t need to make use of this server-side, I’d just use JavaScript objects, which would make this post considerably shorter!)
The Lightning Component
The Lightning component has two sections - the first displays a list of account names and checkboxes for the user to choose accounts, and the second to display details of the chosen accounts:
<aura:component controller="AccountController">
<aura:handler name="init" value="{!this}" action="{!c.doInit}" />
<aura:attribute name="wrappers" type="AccountWrapper" />
<aura:attribute name="accounts" type="Account" />
<span class="big">Choose Accounts</span>
<table>
<tr>
<th class="head">Name</th>
<th class="head">View?</th>
</tr>
<aura:iteration items="{!v.wrappers}" var="wrap">
<tr>
<td class="cell">
<ui:outputText value="{!wrap.acc.Name}" />
</td>
<td class="cell">
<ui:inputCheckbox value="{!wrap.selected}" />
</td>
</tr>
</aura:iteration>
</table>
<button onclick="{!c.getAccounts}">Get Accounts</button>
<br/>
<br/>
<br/>
<span class="big">Selected Accounts</span>
<table>
<tr>
<th class="head">Name</th>
<th class="head">Industry</th>
<th class="head">Website</th>
</tr>
<aura:iteration items="{!v.accounts}" var="acc">
<tr>
<td class="cell">
<ui:outputText value="{!acc.Name}" />
</td>
<td class="cell">
<ui:outputText value="{!acc.Industry}" />
</td>
<td class="cell">
<ui:outputText value="{!acc.Website}" />
</td>
</tr>
</aura:iteration>
</table>
</aura:component>
Note that the AccountWrapper custom class is defined as the type of the wrappers attribute that is used to display the accounts and their checkboxes. This allows me to supply the wrapper classes from the server in the future if for some reason I decide to go that route.
The Lightning Component JavaScript
The controller for the Lightning component contains a couple of methods that delegate to the helper and isn’t that exciting. For those that need the complete picture, its available at this gist.
The helper is much more interesting, but a bit large to drop into this post. It can be found at this gist, but the key aspects are covered below.
The wrapper class instances are created client side, which is really easy in JavaScript, mainly due to its loose typing. I can just define an object instance that contains an account and a selected property. In the snippet below, accs is the collection of accounts returned from the controller at initialisation:
var wrappers=new Array();
for (var idx=0; idx<accs.length; idx++) {
var wrapper = { 'acc' : accs[idx],
'selected' : false
};
wrappers.push(wrapper);
}
cmp.set('v.wrappers', wrappers);
Once the user selects the accounts and presses the getAccounts button, I can iterate the list of wrapper classes and pull the ids of the selected items, which I then turn into the JSON string:
var wrappers=cmp.get('v.wrappers');
var ids=new Array();
for (var idx=0; idx<wrappers.length; idx++) {
if (wrappers[idx].selected) {
ids.push(wrappers[idx].acc.Id);
}
}
var idListJSON=JSON.stringify(ids);
The Lightning Component Styling
In order to make the page a bit more readable, there’s some styling around the component. This is also not particularly interesting but can be found at this gist.
The Application
In order to use this component, I’ve created a simple Lightning application:
<aura:application >
<c:AccountWrappers />
</aura:application>
Opening the application displays the first 10 accounts retrieved from the database, which I can check the associated box to select:
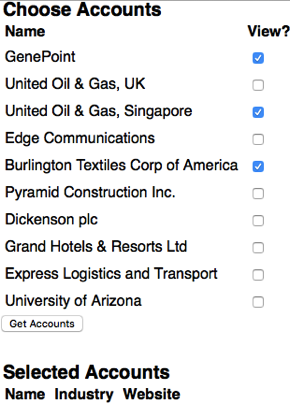
and clicking the Get Accounts button retrieves details of those accounts and displays them:
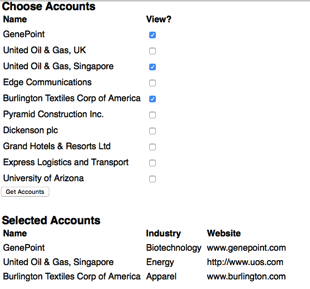
Related Posts